Zgoubidoo’s utility modules¶
Affine geometry using the frame module¶
Module for handling of affine geometry transformations (rotations and translations).
This module provides support for affine geometry transformations, mainly through the Frame class. A typical use case, the one that triggered the development of this module for ::py:module Zgoubidoo, is the problem of placing a sequence with each object being placed with respect to the one preceeding it, with each object being potentially translate or rotated. In such a case, the ::py:module Frame module allows to define a reference frame for each object being placed, with the reference frame of the newly created object using the reference frame of the previous object as a reference frame for its own positioning. The translations and rotations of the object are thus trivially expressed in its own reference frame. The ::py:module Frame module then allows to query the coordinates and rotation information of the object with respect to any other reference frame in the chain of transformations. In particular, the coordinates of the origin of a frame along with its orientation in space, can be obtained with respect to a global (absolute) reference frame.
Example
example TODO
Classes¶
|
A Frame object represents a reference frame for affine geometry transformations (rotations and translations). |
Exception raised for errors in the Frame module. |
|
|
Initialize a Frame with respect to a parent frame. |
Class Inheritance Diagram¶
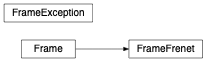
Accelerator physics and relativity calculations using the physics module¶
Zgoubidoo relativistic physics module.
This module provides a collection of functions and classes to deal with relativistic physics computations. This mainly
concerns conversions between kinematic quantities. Full support for units (via pint
) is provided. Additionnally, a
helper class (Kinematics
) provides automatic construction and conversion of kinematics quantities.
Examples
>>> 1 + 1
TODO
Functions¶
|
Converts relativistic beta to magnetic rigidity. |
|
Converts relativistic beta to kinetic energy. |
|
Converts relativistic beta to total energy. |
|
Converts relativistic beta to relativistic gamma. |
|
Converts relativistic beta to momentum. |
|
Converts relativistic beta to relativistic pv. |
|
Converts relativistic beta to range. |
|
Converts magnetic rigidity (brho) to relativistic beta. |
|
Converts magnetic rigidity (brho) to kinetic energy. |
|
Converts magnetic rigidity (brho) to total energy. |
|
Converts magnetic rigidity (brho) to relativistic gamma. |
|
Converts magnetic rigidity (brho) to momentum. |
|
Converts magnetic rigidity (brho) to relativistic pv. |
|
Converts magnetic rigidity (brho) to range. |
|
Converts the kinetic energy to relativistic beta. |
|
Converts kinetic energy to magnetic rigidity (brho). |
|
Converts kinetic energy to total energy |
|
Converts the kinetic energy to relativistic gamma. |
|
Converts kinetic energy to momentum |
|
Converts kinetic energy to relativistic pv. |
|
Converts kinetic energy to proton range in water; following IEC-60601. |
|
Converts total energy to relativistic beta. |
|
Converts total energy to magnetic rigidity (brho). |
|
Converts total energy to kinetic energy. |
|
Converts total energy to relativistic gamma. |
|
Converts total energy to momentum. |
|
Converts total energy to relativistic pv. |
|
Converts total energy to proton range in water; following IEC-60601. |
|
Converts relativistic gamma to relativistic beta. |
|
Converts relativistic gamma to magnetic rigidity. |
|
Converts relativistic gamma to kinetic energy. |
|
Converts relativistic gamma to total energy. |
|
Converts relativistic gamma to momentum. |
|
Converts relativistic gamma to relativistic pv. |
|
Converts relativistic gamma to range (protons only). |
|
Converts momentum to relativistic beta. |
|
Converts momentum to magnetic rigidity (brho). |
|
Converts momentum to kinetic energy. |
|
Converts momentum to total energy. |
|
Converts momentum to relativistic gamma. |
|
Converts momentum to relativistic pv. |
|
Converts momentum to proton range in water; following IEC-60601. |
|
Converts proton range in water to relativistic beta. |
|
Converts proton range in water to magnetic rigidity (brho). |
|
Converts proton range in water to kinetic energy following IEC60601. |
|
Examples |
|
Converts proton range in water to relativistic beta. |
|
Converts proton range in water to momentum. |
|
Converts proton range in water to relativistic pv. |
Classes¶
|
|
Exception raised for errors in the Zgoubidoo physics module. |
Class Inheritance Diagram¶
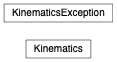
Polarity type system¶
Type system for polarities.
Examples
The conversion to numeric types can be used for easily in numerical expressions:
>>> gradient = int(HorizontalPolarity) * 3.1415; gradient
>>> 3.1415
One major use case consists in type-hinting functions (shown here with default value argument):
>>> def foo(polarity: PolarityType = HorizontalPolarity): pass